We will see how we can add our data from WordPress WooCommerce to Firebase Firestore using Firebase cloud functions.
Set up a local WordPress site
You will need to set up a server and a database in order to get WordPress up and running. There are different options available you can follow my other article on setting up WordPress locally.
Install WordPress on localhost XAMPP.
Set up WooCommerce store
You will need to set up your WooCommerce store.
You can follow my other article on setting WooCommerce plugin with WordPress.
Setting WooCommerce with WordPress and creating first product.
Set up Firebase
You will need to set up firebase cloud function and firestore.
For using cloud function, you need to be on blaze plan (pay as you go). Don’t worry you won’t be charged anything initially as it provides free limit every month.
STEP 1:
Go to Firebase console.

STEP 2:
Create a new project. Click on Add project button.

STEP 3:
Give your project a unique name.
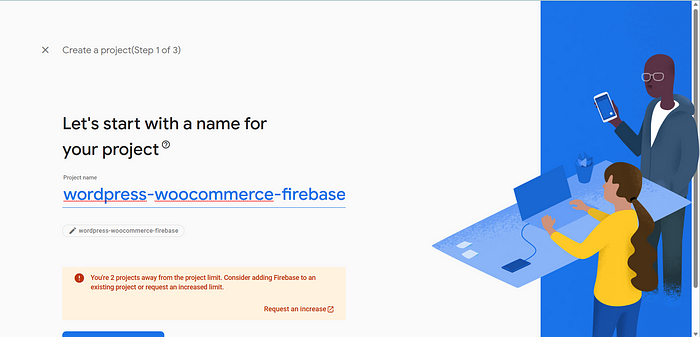
STEP 4:
Enable google analytics for this project and press continue.

STEP 5:
Choose or create a google analytics account.

STEP 6:
Project has been created successfully. Press continue.

STEP 7:
Now click on Functions in the sidebar. It will show you to upgrade your project if you are not already on the blaze plan.

Upgrade your Firebase account to Blaze plan
Now let’s upgrade our account to blaze plan together. Press continue button.
You can also click on see full plan details to view the pricing structure.

STEP 1:
Pricing structure for Firebase cloud functions.

STEP 2:
Now, you need to select your country and press confirm.

STEP 3:
Enter your business name (can be anything) and add your card details.

STEP 4: (Optional)
You can set up a billing budget. If the cost approaches or exceeds the amount you will get an email.

STEP 5:
Now click on purchase.

STEP 6:
Congratulations you have successfully upgraded to blaze plan.

Firebase Cloud Function
Now click on get started.

STEP 1
You need to have node installed on your system.
You can download it from here. After downloading and installing node open your command prompt and run this command.
npm install -g firebase-tools


STEP 2:
Now, first run the login command if you aren’t logged in. Then the firebase init command.
firebase login
firebase init


Firebase login command will open a browser window. Login from there. Select the account with which you created the project for using cloud functions.

Login success, now you can close this window.

Now run the firebase init command.
Press Y and enter to proceed.

STEP 3:
Move up and down using arrow keys and select Functions by pressing spacebar and press enter.

STEP 4:
Now select use an existing project by pressing enter.

STEP 5:
Move up and down using arrow keys and press enter on the project name you created on firebase for using cloud functions.

STEP 6:
Now enter the following information
What language would you like to write Cloud Functions? JavaScript
Do you want to use EsLint to catch probable bugs and enforce style? No
Do you want to install dependencies with npm now? Yes
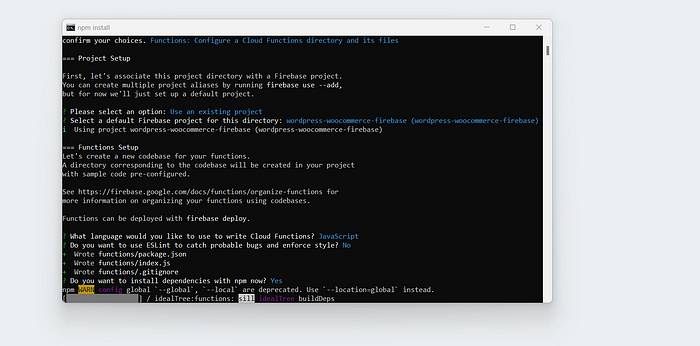
STEP 7:
Firebase initialization has been completed. Open the functions folder created in any code editor of your choice. I am using vscode.

STEP 8:
Open the index.js file.
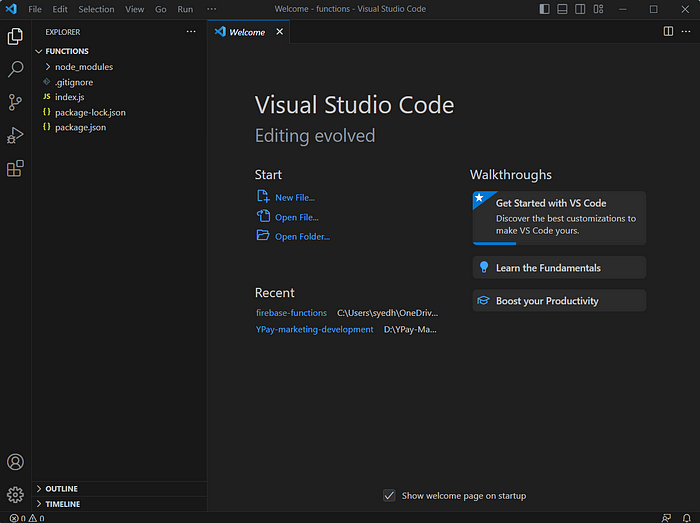
STEP 9:
Replace the following code in the file with this.
/**
* Import function triggers from their respective submodules:
*
* const {onCall} = require("firebase-functions/v2/https");
* const {onDocumentWritten} = require("firebase-functions/v2/firestore");
*
* See a full list of supported triggers at https://firebase.google.com/docs/functions
*/
const {onRequest} = require("firebase-functions/v2/https");
const logger = require("firebase-functions/logger");
// / The Firebase Admin SDK to access Firestore.
const admin = require('firebase-admin');
admin.initializeApp();
// Create and deploy your first functions
// https://firebase.google.com/docs/functions/get-started
exports.addOrders = onRequest(async (request, response) => {
logger.info("Hello logs!", {structuredData: true});
const writeOrderResult = await admin.firestore().collection('orders').add({...request.body});
response.send("Order created successfull!");
});

STEP 10:
Save the index.js file and run the following command in cmd.
firebase deploy
You might get an error.
User code failed to load. Cannot determine backend specification.

STEP 11:
The error is, when you initialize Firebase, it uses the latest Nodejs version (currently 18).
To remove the error open package.json file. And add the node version installed on your system. (For me it’s 16)
"engines": {
"node": "16"
}

Run the firebase deploy command again.

STEP 12:
Now if you go to firebase cloud function dashboard, you can see that addOrders function has been added successfully.
Copy the URL under Trigger.

Set up Firebase Firestore
STEP 1:
Go to Cloud Firestore from Firebase console and click on create database.

STEP 2:
Select start in Test mode and click next.
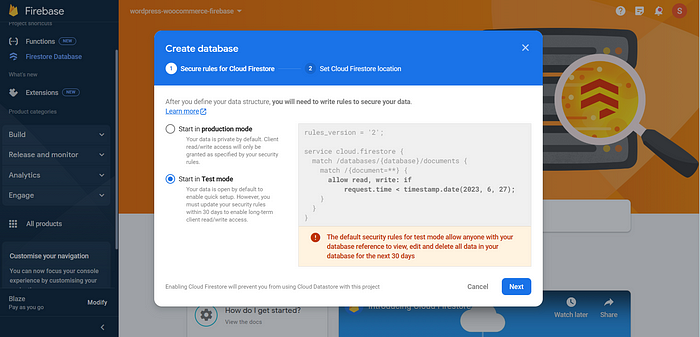
STEP 3:
Select a location for your database and click on enable.

STEP 4:
Congratulations you have successfully set up your Firestore database.

Now, the final part of connecting Firebase with WordPress WooCommerce
STEP1:
Go to WordPress Dashboard and click on WooCommerce settings in the sidebar. And click on Advanced tab.
Click on Webhooks and add the following details
Name: addOrder (can be any)
Status: Active
Topic: Order created (can select any in the list. I have selected order created. This webhook will be fired when an order is created.)
Delivery URL: https://addorders-fmaeoxddgq-uc.a.run.app (which you copied from Firebase Cloud Function console.)
API Version: WP REST API Integration v3

STEP 2:
Now let’s test it out.
Go to your website and on shop page, press add to cart.

Click on checkout on Cart’s page.

STEP 3:
Enter your billing information, and submit.

STEP 4:
Order has been successfully created on WooCommerce.

STEP 5:
Head over to firebase firestore, to view your order data.

Congratulations you have successfully set up WooCommerce WordPress plugin with Firebase.